0-π Qubit
In this notebook, we try to reproduce the result of the “Coherence properties of the 0-π qubit” paper.
Introduction
Our goal is to reproduce the enegry spectrum and eigenfunctions that Groszkowski2018 is calculated for the \(0-\pi\) qubit. The diagram of the circuit is
We choose the parameter set 3 for the circuit from the following table of the paper:
Circuit Description
Firstly, we import the SQcircuit and the relavant libraries
[1]:
import SQcircuit as sq
import matplotlib.pyplot as plt
import numpy as np
We define the single inductive loop of the circuit via Loop
class
[2]:
loop1 = sq.Loop()
The elements of the circuit can be defined via Capacitor
, Inductor
, and Junction
classes in SQcircuit, and to define the circuit, we use the Circuit
class.
[3]:
# define the circuit ’s elements
C = sq.Capacitor(0.15, "GHz")
CJ = sq.Capacitor(10, "GHz")
JJ = sq.Junction(5, "GHz", loops=[loop1])
L = sq.Inductor(0.13, "GHz", loops=[loop1])
# define the circuit
elements = {(0, 1): [CJ, JJ],
(0, 2): [L],
(0, 3): [C],
(1, 2): [C],
(1, 3): [L],
(2, 3): [CJ, JJ]}
zrpi = sq.Circuit(elements)
By creating an object of Circuit
class, SQcircuit systematically finds the correct set of transformations and basis to make the circuit ready to be diagonalized.
Before setting the truncation numbers for each mode and diagonalizing the Hamiltonian, we can gain more insight into our circuit by calling the description()
method. This prints out the Hamiltonian and a listing of the modes, whether they are harmonic or charge modes, and the frequency for each harmonic in GHz (the default unit). Moreover, it shows the prefactors in the Josephson junction part of the Hamiltonian \(\tilde{\textbf{w}}_k\), which helps find the modes decoupled from the
nonlinearity of the circuit.
[4]:
zrpi.description()
The above output shows that the first mode of the zrPi
circuit is a harmonic mode with the natural frequency of 3.22 in the frequency unit of SQcircuit (which is GHz in default), and the second mode is a charge mode.
To determine the size of the Hilbert space, we specify the truncation number for each circuit mode via set_trunc_nums()
method. Note that this is a necessary step before diagonalizing the circuit.
[5]:
zrpi.set_trunc_nums([35, 6])
Circuit Spectrum
To generate the spectrum of the circuit, firstly, we need to change and sweep the external flux of loop1
by the set_flux()
method. Then, we need to find the eigenfrequencies of the circuit that correspond to that external flux via diag()
method. The following lines of code find the spec
a 2D NumPy array so that each column of it contains the eigenfrequencies with respect to its external flux.
[6]:
# external flux for sweeping over
phi = np.linspace(0,1,100)
# spectrum of the circuit
n_eig=5
spec = np.zeros((n_eig, len(phi)))
for i in range(len(phi)):
# set the external flux for the loop
loop1.set_flux(phi[i])
# diagonlize the circuit
spec[:, i], _ = zrpi.diag(n_eig)
[7]:
plt.figure()
for i in range(n_eig):
plt.plot(phi, spec[i,:]- spec[0,:])
plt.xlabel(r"$\Phi_{ext}/\Phi_0$", fontsize=13)
plt.ylabel(r"$f_i-f_0$[GHz]", fontsize=13)
plt.show()
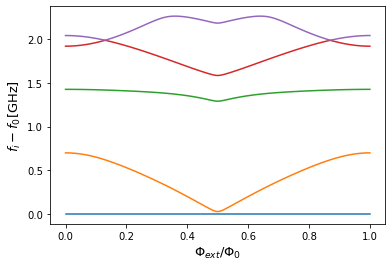
The next cell shows the spectrum from the figure 4 of the paper, which is the same spectrum that SQcircuit calculated.
Eigenfunctions
We generate the eigenfunction in the phase space by eig_phase_coord()
method for the ground and first excited state. To calculate the eigenfunction at \(\Phi_{ext} = 0.9\Phi_0\) similar to paper, we set back the flux of our loop to \(0.9\Phi_0\) and diagonalize the zrPi
again.
[8]:
loop1.set_flux(0.9)
zrpi.set_trunc_nums([35, 11])
_, _ = zrpi.diag(n_eig)
[9]:
# create a range for each mode
phi = np.pi*np.linspace(-1,1,100)
theta = np.pi*np.linspace(-0.5,1.5,100)
# the ground state
state0 = zrpi.eig_phase_coord(0, grid=[phi, theta])
# the first excited state
state1 = zrpi.eig_phase_coord(1, grid=[phi, theta])
[10]:
plt.figure(figsize=(5, 2))
plt.pcolor(phi, theta, np.abs(state0)**2,cmap="binary",shading='auto')
plt.xlabel(r'$\phi$')
plt.ylabel(r'$\theta$')
[10]:
Text(0, 0.5, '$\\theta$')
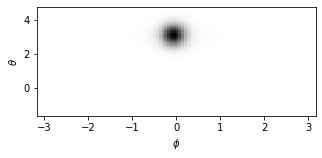
[11]:
plt.figure(figsize=(5, 2))
plt.pcolor(phi, theta, np.abs(state1)**2,cmap="binary",shading='auto')
plt.xlabel(r'$\phi$')
plt.ylabel(r'$\theta$')
[11]:
Text(0, 0.5, '$\\theta$')
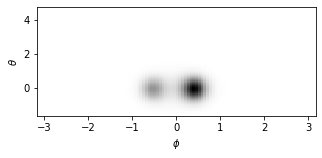